As the semester is coming to a close in console I chose to create a basic mobile application in Java. I have done some Java in the past however never for Mobile device. So looking at this I decided to try using Android Studio which had some reviews and go through the first tutorial. First looking at how easily the program linked with my nexus was great. Many applications I have used they have ran into some step where my computer and the nexus wouldn't connect and this walkthrough went through great.
From there I opened and created my first project. This is great between the XML and Java, they work so smooth together it is easy to define strings in the XML and than use them with your program using @ after defining it. I went through the basic Activity screen via the parent process so that one would call the other.
This took a bit and some steps on the walk through were left out or confusing, but the application went smooth and at the end got a basic app, that takes the text of the user and than outputs it into the other activity afterwards. Overall this was very fun and I see myself continuing the tutorial both for understanding Mobile development from something other than Unity and Action-script (All I have done in the past.) Also it will help me develop better Java skills, which I have written very little Java as a whole, and have heard many good things about the language.
Networking 2013
Tuesday, April 28, 2015
Physics Rigid Body
Wednesday, April 8, 2015
Alibi Generation
In one of my classes I took on using an AI application that would create Alibis at run time. When looking into the process itself is seem to use mostly statistic Algorithm to choose which alibi to create. So for this it mean saving a lot of numbers. The equation itself is Y = { 1/[ σ * sqrt(2π) ] } * e-(x - μ)2/2σ2 . . Y is the percent chance at X and the sigma (o looking) is the standard deviation from the mean which is the where μ is the mean, and σ is the standard deviation. The standard deviation is a normal amount above and below the mean value it would be alright with. So with this we see that we get a single Y value at X. Which means to get a true probability we need to do it many more times. For my generation I go up til I hit the Mean + Deviation. So 25 Characters with 5 deviation I go from 0 - 30 to calculate the first chance of creation. From that point You do from current to that max. So if 5 people were already alive to check the percentage of any number of people between 5 and 30. You would check for 6 - 30. Adding each one would give you a proper percent.
public const float e = 2.71828f;
//cumlative probabilty of ans < randVariable based on the mean and standDev
//so if you wanted to know how long a light bulb would last for 1200 hours or less
//the mean would be 1000 and dev would be 100
// you would use CumlativeNormalDis(1200,1000,100) the percentage would be 97
static public float NormalDis(float randVariable, float mean, float standDev)
{
float y = (float)1.0f / (standDev * (float)Math.Sqrt(2.0f * Math.PI));
float z = -1 * (((randVariable - mean) * (randVariable - mean)) / (2.0f * standDev * standDev));
float x = e;
x = (float)Math.Pow(x, z);
y = y * x;
return y;
}
//--------------------------------------------------------------------------------------------
static public float CumlativeNormalDis(float randVariable, float mean, float standDev)
{
float ans = 0;
for(int i = 0; i < randVariable;i++)
{
ans += NormalDis(i, mean, standDev);
}
return ans * 100;
}
This is specifically my two function in order to create the percentage from 0 - 100 cumulatively. As well I leave a comment above on how to use it. You can see how this would be time consuming. Running this equation over and over just to get a percent between 0 - 1. Now imagine with everything on top of this creating full alibis for a character would take time. Luckily you would be doing a sector or even just a current location. You would want to keep the alibis to a smaller amount. Never try to create 1000 full alibis for all character.
I chose full alibi above, when I use full I mean not only are we deciding how this certain character would look like, but some background some likes dislikes, this way if we allow communication between the player and say this NPC, he would actually know why he was standing where he was. He choose lets say to grab a coffee from place X over place Y and the player watched him walk from right next to the other place all the way to X. With just random pathing there is no reason for him other than looking good to make him walk. However if he has an alibi that states he loves the scones from this place and hates the coffee from the other he actually has a reason(an alibi) to go through the extra walking.
Now if I was to put this into a full game I envision, some would need to be created live like why they are pathing to a location. However some of the background looks, and some likes and dislikes could be created before and left in groups. This way you have a couple option to put in an area. So each say grid space you would create at once has 3-4 variations saved. This way they were run before the game was even shipped and this would save a lot of time and allow that to be used in the game. However the use during is still feasible and the ability to take these alibis later and populate an area to a reasonable amount not having too many or too few people at one time can help trick a player.
As well very detailed alibis help create this layer of confusion, was this character built, have a seen him/her before, Or is this a new character, the player wouldn't be able to tell unless he remember and talked to every player he has ever met. This also allows a proper flow of character that look like they belong somewhere in the game. It keeps characters that don't look like they belong in lets say a library from a library and those who would be there go there. It adds a nice level of belief to the player when he walks through an open world. Overall I believe using these alibis is extremely helpful to a player, to fully immerse in the world. When they talk to anyone and they have the alibis and background on their life it looks like the world is real. If you walked up and got a generic hello for hundredth time it can break the immersion. You as a developer can go as deep into the alibi pool as possible even giving details on work, and personal history of the character in the world. You can go as little as a suit or tee-shirt.
public const float e = 2.71828f;
//cumlative probabilty of ans < randVariable based on the mean and standDev
//so if you wanted to know how long a light bulb would last for 1200 hours or less
//the mean would be 1000 and dev would be 100
// you would use CumlativeNormalDis(1200,1000,100) the percentage would be 97
static public float NormalDis(float randVariable, float mean, float standDev)
{
float y = (float)1.0f / (standDev * (float)Math.Sqrt(2.0f * Math.PI));
float z = -1 * (((randVariable - mean) * (randVariable - mean)) / (2.0f * standDev * standDev));
float x = e;
x = (float)Math.Pow(x, z);
y = y * x;
return y;
}
//--------------------------------------------------------------------------------------------
static public float CumlativeNormalDis(float randVariable, float mean, float standDev)
{
float ans = 0;
for(int i = 0; i < randVariable;i++)
{
ans += NormalDis(i, mean, standDev);
}
return ans * 100;
}
This is specifically my two function in order to create the percentage from 0 - 100 cumulatively. As well I leave a comment above on how to use it. You can see how this would be time consuming. Running this equation over and over just to get a percent between 0 - 1. Now imagine with everything on top of this creating full alibis for a character would take time. Luckily you would be doing a sector or even just a current location. You would want to keep the alibis to a smaller amount. Never try to create 1000 full alibis for all character.
I chose full alibi above, when I use full I mean not only are we deciding how this certain character would look like, but some background some likes dislikes, this way if we allow communication between the player and say this NPC, he would actually know why he was standing where he was. He choose lets say to grab a coffee from place X over place Y and the player watched him walk from right next to the other place all the way to X. With just random pathing there is no reason for him other than looking good to make him walk. However if he has an alibi that states he loves the scones from this place and hates the coffee from the other he actually has a reason(an alibi) to go through the extra walking.
Now if I was to put this into a full game I envision, some would need to be created live like why they are pathing to a location. However some of the background looks, and some likes and dislikes could be created before and left in groups. This way you have a couple option to put in an area. So each say grid space you would create at once has 3-4 variations saved. This way they were run before the game was even shipped and this would save a lot of time and allow that to be used in the game. However the use during is still feasible and the ability to take these alibis later and populate an area to a reasonable amount not having too many or too few people at one time can help trick a player.
As well very detailed alibis help create this layer of confusion, was this character built, have a seen him/her before, Or is this a new character, the player wouldn't be able to tell unless he remember and talked to every player he has ever met. This also allows a proper flow of character that look like they belong somewhere in the game. It keeps characters that don't look like they belong in lets say a library from a library and those who would be there go there. It adds a nice level of belief to the player when he walks through an open world. Overall I believe using these alibis is extremely helpful to a player, to fully immerse in the world. When they talk to anyone and they have the alibis and background on their life it looks like the world is real. If you walked up and got a generic hello for hundredth time it can break the immersion. You as a developer can go as deep into the alibi pool as possible even giving details on work, and personal history of the character in the world. You can go as little as a suit or tee-shirt.
Tuesday, March 31, 2015
Physics Rods, Cables, and Springs
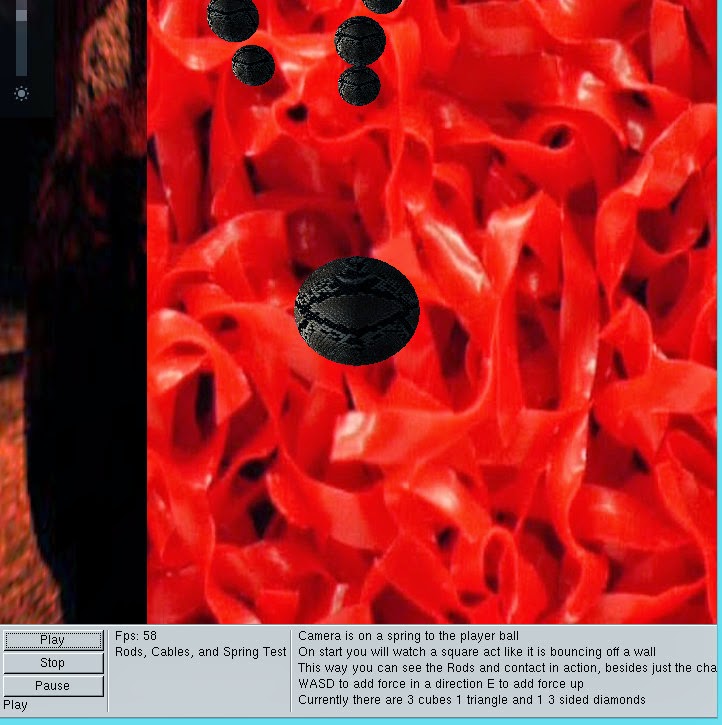
Currently I have completed a simulation for Rods, Cables, Springs, and contact generators at the particles level. I noticed during the quick implementation of OpenGL and the graphics system built on top caused some frame-rate issues. I needed to go back and re-factor the way the images got processed and streamlined it into a class, which is more efficient for numerous reasons. In these pictures the player being the ball is following around a cube, put together of Rods, so that it doesn't lose its shape.
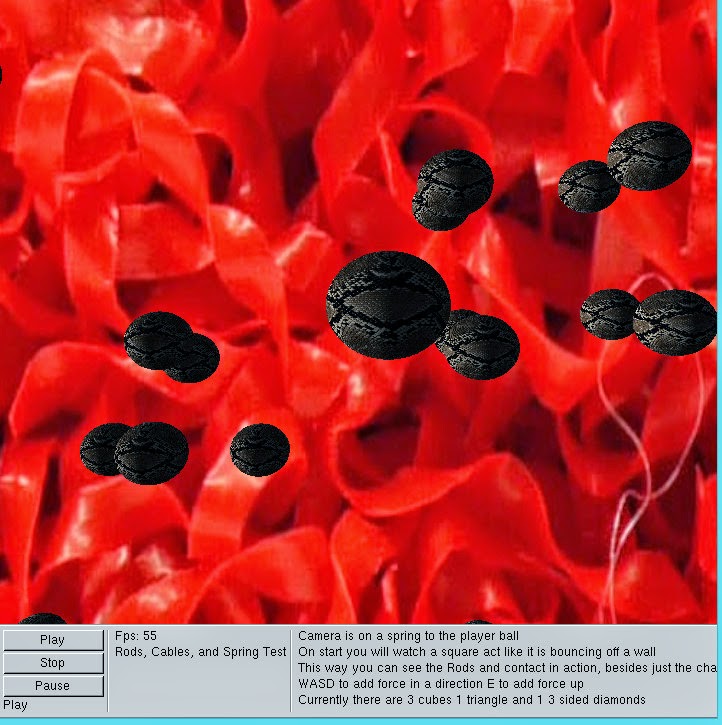
Above you see a diamond like shape that has fallen because it has 3 sides in the middle not 4. In a perfect simulation a 4 sided diamond would stand perfectly on a particle because all forces would hit equilibrium once it landed on the ground. As you look at the pictures the camera is slightly off of where the ball is and it's because currently the camera is based off of a physics object attached to the players. So sudden moves and stopping causes a little elastic motion for the player. In the bottom you can see the wall which all physics object bounce off of including the camera. This iteration I also took the time to set it up by game objects which render, and physics objects which just update collide etc. This also increased performance, and allowed the camera to have physics without having to fake render anything. Current shapes that can be rapidly created by my physics implementation are cubes, triangular pyramids, and triangular diamonds. These can be quickly created by the level, just by creating and setting a center point and the width of the total object. It will figure the rest out and easily set-up cables, rods, or springs depending on what you would have wanted.
Monday, February 16, 2015
Physics Solar System
The video software states that its running at one FPS, but that text is just an error with the video. It would be a lot worse at one FPS, because I have a DPF which is days per second because I have a game timer in my simulation. This way a loss in frame rate doesn't slow the simulation down itself. So when my when an update occurs it runs through the physics system, that many times between 0 and 150 which are the min and max. This way it does the math day by day instead of just doing it one large jump which would cause a lot of errors, both floating points and getting correct velocity and acceleration for each section. Since I am using euler physics there are errors overtime, some calculations were done before putting it into the system so it would round less and I could use doubles for maths, but save as floats. However there is some rounding and just using the general gravity does not produce a perfect simulation. If I was going to take this farther I would use Keplers' laws of planetary motion to create a better simulation, but it would have required a lot more time than I had.
Tuesday, April 29, 2014
Abyssi Production 2 Project
Monday, February 24, 2014
Sights and Sound
In production 1 of early times I worked on another project that went extremely well and the group I feel worked flawlessly with each other. Again I worked with a another programmer. This time my other Programmer was Alex Beauchesne, my designer was Devon Case, and Artists were Kristin Darling and Caitlin Coon For this project when something was needed or necessary our group never had a problem meeting or talking at all times of the day, which really helped this game which had just a few weeks get extremely far and work very well. We were told to make an educational game so what we went was, teaching children how to solve puzzles using another character which you switch between and eventually would be two player and each would help and control one of them. Each had abilities which the other didn't and these were strengths the other lacked. For this game we had a character who had earmuffs stuck to his air so he was technically deaf and the other had a bandanna over her eyes, so she was technically blind and they used the other member sense the lacked to make it through the course. The game progressively moves the player forward and has introductions and teaches the player how to use each player through the first few levels then requires the player to use the past until you get to a real level with no new activities and you are put to the test.
Subscribe to:
Posts (Atom)